If you’re working with Docker and need a container that runs indefinitely without any predefined tasks, using the sleep infinity command is a simple and effective approach. This article will walk you through creating a minimal Dockerfile that ensures your container stays active indefinitely.
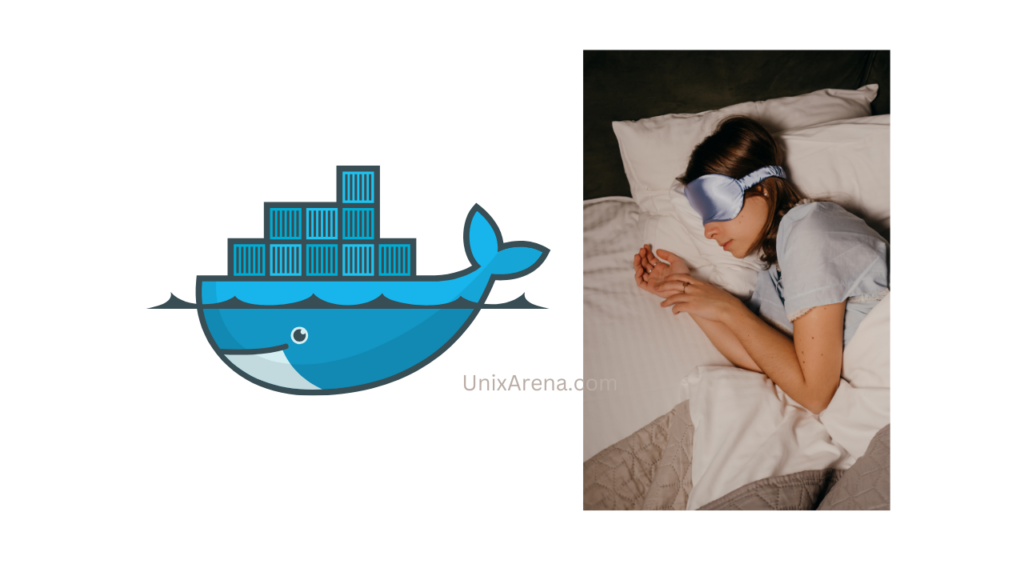
Why Use sleep infinity in Docker?
By default, Docker containers stop running when the main process in the container exits. If you’re using a container to debug, test, or keep it running as a placeholder, having a process like sleep infinity is a clean solution. It prevents the container from exiting prematurely.
Step-by-Step Guide to Create a Dockerfile with sleep infinity
Follow these simple steps to set up a lightweight Docker container that stays active indefinitely.
1. Create a New Directory for Your Dockerfile
Start by creating a dedicated directory for your project:
ux$ mkdir docker-sleep
ux$ cd docker-sleep
2. Create the Dockerfile
Use your favorite text editor (like nano or vim) to create a Dockerfile:
ux$ vi Dockerfile
3. Write the Dockerfile Contents
Here’s the Dockerfile that keeps your container running indefinitely:
# Step 1: Use a lightweight base image
FROM alpine:latest
# Step 2: Set up the working directory (optional)
WORKDIR /app
# Step 3: Keep the container running indefinitely
CMD ["sleep", "infinity"]
Breakdown of the Dockerfile:
• FROM alpine:latest: Uses Alpine Linux as the base image because it’s lightweight, fast, and has minimal overhead.
• WORKDIR /app: Sets the working directory to /app (optional).
• CMD [“sleep”, “infinity”]: Runs the sleep infinity command, ensuring the container never stops until manually terminated.
4. Build and Run the Docker Container
Once your Dockerfile is ready, follow these steps to build and run the container.
Build the Docker Image
Run the following command to build the image and tag it (e.g., sleep-infinity):
ux$ docker build -t sleep-infinity .
Run the Docker Container
Use the docker run command to start a container from the image:
ux$ docker run -d sleep-infinity
The -d flag runs the container in detached mode, allowing it to run in the background.
Verifying the Container is Running
To confirm that your container is running, use the following command:
ux$ docker ps
You should see an entry similar to this:
CONTAINER ID IMAGE COMMAND STATUS PORTS NAMES
ux-sleep sleep-infinity "sleep infinity" Up 15 minutes practical_lamport
Use Cases for sleep infinity
1. Debugging: Keep the container running while you inspect its filesystem or logs.
2. Placeholder Containers: Use it as a base to add further customizations later.
3. Testing and Development: Useful for scenarios where a running container is required without actual processes.
Conclusion
Using sleep infinity in a Dockerfile is a simple yet powerful way to keep your containers running indefinitely. This is especially useful for debugging, testing, or setting up placeholder containers. By following the steps in this guide, you can quickly create and run a lightweight container that stays active as long as needed.
If you found this guide helpful, feel free to share it or leave a comment below. Happy Dockerizing! 🚀
Leave a Reply